Geolocation クラスについて
■Geolocation クラスについて
Geolocation クラスを使用すると、ユーザーの位置情報を取得することができます。
高度や、経度緯度、方角などを取得することができます。
■ジオロケーションに対応しているか調べる
デバイスがジオロケーション機能の利用が可能か調べるには、Geolocation.isSupported プロパティを調べます。
true であれば、利用可能です。
ジオロケーションが利用可能か調べる
import flash.text.TextField;
import flash.sensors.Geolocation;
// テキストフィールドを作成
var text_field:TextField = new TextField();
text_field.x = 10;
text_field.y = 10;
text_field.width = stage.stageWidth - 20;
text_field.height = 20;
text_field.border = true;
stage.addChild(text_field);
text_field.text = "ジオロケーションのサポート:" + Geolocation.isSupported;
■アンドロイドでジオロケーション機能を使用する
「AIR for Android」でジオロケーション機能を使用するには、以下のパーミッション設定を追加する必要があります。
権限名 | 説明 |
ACCESS_FINE_LOCATION | GPS にアクセスする(高品質) |
ACCESS_COARSE_LOCATION | WiFi やセルラーのネットワーク位置情報にアクセスする(低品質) |
■Adobe Flash による設定方法
「AIR for Android 設定」の「権限」タブにある、「ACCESS_FINE_LOCATION」と「ACCESS_COARSE_LOCATION」のチェックを有効にします。
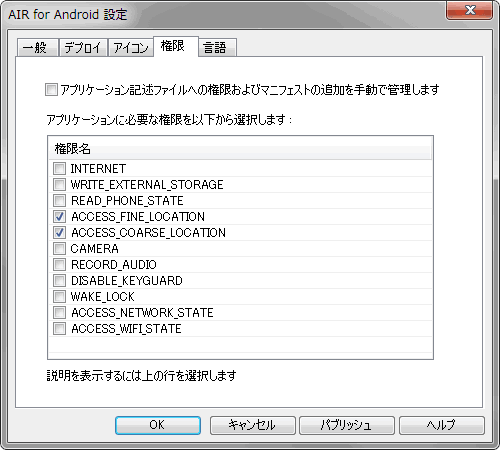
■アプリケーション記述ファイルを直接編集する方法
アプリケーション記述ファイルに、以下のパーミッション設定を追加します。
アプリケーション記述ファイルに autoOrients タグを追加する
<?xml version="1.0" encoding="UTF-8" standalone="no" ?>
<application xmlns="http://ns.adobe.com/air/application/2.0">
~略~
<android>
<manifestAdditions>
<![CDATA[<manifest>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"/>
</manifest>]]>
</manifestAdditions>
</android>
</application>
■ジオロケーションオブジェクトを作成する
new 演算子を使って、Geolocation クラスをインスタンス化します。
ジオロケーションオブジェクトを作成する
import flash.sensors.Geolocation;
// ジオロケーションオブジェクトを作成
var geo_location:Geolocation = new Geolocation();
■ユーザーがジオロケーションをミュートしているか調べる
ユーザーの設定により、ジオロケーションが利用停止状態か調べるには、muted プロパティを調べます。
true であれば、利用できません。
ジオロケーションがミュート設定か調べる
import flash.text.TextField;
import flash.sensors.Geolocation;
// ジオロケーションに対応している
if(Geolocation.isSupported){
// テキストフィールドを作成
var text_field:TextField = new TextField();
text_field.x = 10;
text_field.y = 10;
text_field.width = stage.stageWidth - 20;
text_field.height = 20;
text_field.border = true;
stage.addChild(text_field);
// ジオロケーションオブジェクトを作成
var geo_location:Geolocation = new Geolocation();
text_field.text = "ジオロケーションのミュート設定:" + geo_location.muted;
}
■ユーザーがジオロケーションの設定を変更したか監視する
ユーザーが、ジオロケーションの設定を変更したか監視するには、StatusEvent.STATUS イベントを使用します。
muted プロパティに変更があるたびに、登録した関数が実行されます。
ユーザーが、ジオロケーションの設定を変更したか監視する
import flash.text.TextField;
import flash.sensors.Geolocation;
import flash.events.StatusEvent;
// ジオロケーションに対応している
if(Geolocation.isSupported){
// テキストフィールドを作成
var text_field:TextField = new TextField();
text_field.x = 10;
text_field.y = 10;
text_field.width = stage.stageWidth - 20;
text_field.height = 20;
text_field.border = true;
stage.addChild(text_field);
// ジオロケーションオブジェクトを作成
var geo_location:Geolocation = new Geolocation();
// muted プロパティに変更があるたびに実行されるイベント
geo_location.addEventListener(StatusEvent.STATUS , function (e:StatusEvent):void{
text_field.text = "ジオロケーションのミュート設定:" + geo_location.muted;
});
}
位置情報を取得する
サンプルをダウンロード
■位置情報を取得する
位置情報を取得するには、GeolocationEvent.UPDATE イベントを使用します。
引数から、GeolocationEvent オブジェクトが得られます。
■GeolocationEvent クラスのプロパティ
GeolocationEvent クラスには、以下のプロパティがあります。
プロパティ名 | 型 | 説明 |
latitude | Number | 緯度(単位:角度) |
longitude | Number | 経度(単位:角度) |
altitude | Number | 高度(単位:メートル) |
horizontalAccuracy | Number | 水平精度(単位:メートル) |
verticalAccuracy | Number | 垂直精度(単位:メートル) |
speed | Number | 速度(単位:秒速メートル m/s) |
heading | Number | 方角(単位:角度、真北が 0度) |
■取得できる情報について
デバイスに古い位置情報が残っている場合、リスナーを登録したタイミングで、すぐにその古い情報が得られるようです。
つまり、1回目に得られる情報は、現在の位置ではなく、古い位置である可能性があります。
また、デバイスによっては、2回目以降の通知は滅多に発生しないという場合もあるようです。
■使用例
イベントの設定例です。
ジオロケーションから位置情報を取得する
import flash.text.TextField;
import flash.sensors.Geolocation;
import flash.events.GeolocationEvent;
// ジオロケーションに対応している
if(Geolocation.isSupported){
// テキストフィールドを作成
var text_field:TextField = new TextField();
text_field.x = 10;
text_field.y = 10;
text_field.width = stage.stageWidth - 20;
text_field.height = 200;
text_field.border = true;
stage.addChild(text_field);
// ジオロケーションオブジェクトを作成
var geo_location:Geolocation = new Geolocation();
// 位置情報を取得するイベント
geo_location.addEventListener(GeolocationEvent.UPDATE , function (e:GeolocationEvent):void{
var str:String = "";
str += "緯度:" + e.latitude + "\n";
str += "経度:" + e.longitude + "\n";
str += "高度:" + e.altitude + "\n";
str += "水平精度:" + e.horizontalAccuracy + "\n";
str += "垂直精度:" + e.verticalAccuracy + "\n";
str += "速度:" + e.speed + "\n";
str += "方角:" + e.heading + "\n";
str += "タイムスタンプ:" + e.timestamp + "\n";
text_field.text = str;
});
}
■位置情報の取得時間隔を変更する
GeolocationEvent.UPDATE イベントの実行時間隔を変更するには、setRequestedUpdateInterval() メソッドを使用します。
引数に、数値(単位:ミリ秒)を指定します。
0 以下の数値を指定するとエラーとなります。
必ずしも指定した時間隔でイベントが実行されるとは限りません。
バッテリー節約時など、場合によっては変化することがあります。
位置情報の取得時間隔を変更する
import flash.text.TextField;
import flash.sensors.Geolocation;
import flash.events.GeolocationEvent;
// ジオロケーションに対応している
if(Geolocation.isSupported){
// テキストフィールドを作成
var text_field:TextField = new TextField();
text_field.x = 10;
text_field.y = 10;
text_field.width = stage.stageWidth - 20;
text_field.height = 200;
text_field.border = true;
stage.addChild(text_field);
// ジオロケーションオブジェクトを作成
var geo_location:Geolocation = new Geolocation();
// 位置情報の取得時間隔を変更(単位:ミリ秒)
geo_location.setRequestedUpdateInterval(1000);
// 位置情報を取得するイベント
geo_location.addEventListener(GeolocationEvent.UPDATE , function (e:GeolocationEvent):void{
text_field.text = "タイムスタンプ:" + e.timestamp;
});
}